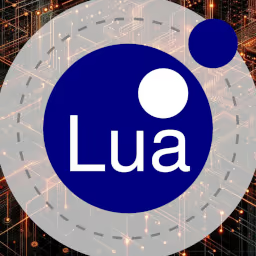
Lua Language Overview
Lua is a lightweight, high-performance scripting language known for its simple syntax and efficient execution. Like Python, Lua is dynamically typed, meaning variables do not need explicit type declarations, making both languages easy to write and flexible. Lua's syntax is clean and concise, with similarities to Python in its handling of loops, conditionals, and functions, though Lua often requires fewer lines of code for basic operations.
DISCLAIMER: The majority of this article was generated from ChatGPT prompts, and curated/edited by a human being.
What is Lua Used For
Lua is a versatile scripting language widely used in game development, particularly for 2D games with frameworks like LÖVE2D. Its lightweight design and easy integration with C and other programming languages make it ideal for embedding in applications, enabling developers to extend functionality and customize behavior. Additionally, Lua is popular in fields such as web development, where it powers server-side applications with frameworks like OpenResty, and in embedded systems for IoT devices due to its efficiency and small footprint. Its flexibility also allows it to be used in data processing, configuration scripting, and as a general-purpose language in various applications.
Why is Lua Used in Games
Lua is widely used in game development for several compelling reasons:
- 1. Performance: Lua is designed to be lightweight and efficient, making it ideal for game engines where performance is crucial. Its execution speed is competitive, allowing for real-time scripting without significant overhead.
- 2. Ease of Integration & Embedding: Lua can be easily embedded in applications written in C, C++, or other languages, making it a popular choice for game engines. This allows developers to extend game functionality and customize behavior without recompiling the entire application.
- 3. Flexible & Dynamic Typing: Lua's dynamic typing allows for rapid prototyping and iterative development, which is essential in game development where design and mechanics often evolve during the process.
- 4. Ease of Learning and Use: Lua has a straightforward and readable syntax, making it accessible for developers, designers, allowing even non-programmers to use it effectively. This ease of use encourages collaboration between developers and other team members.
- 5. Cross-Platform Compatibility: Lua is highly portable and can run on various platforms, making it suitable for multi-platform game development. Developers can use the same Lua scripts across different devices with minimal adjustments.
Lua's popularity in the industry is evidenced by its use in many well-known game engines and titles, enabling developers to create rich and engaging gaming experiences.
How to Install Lua
Visit Lua's downloads page to get started.
How to Install Lua on Windows
To install Lua on Windows, you will need to either compile an executable from the source code, or download a pre-compiled SourceForge binary.
How to Install Lua on macOS
You can get started with Lua on macOS using Homebrew. Use the following brew command to install it:
brew install lua
Building Lua from Source
On a UNIX-like machine, like macOS or Linux, you can compile it from source using make by doing something like this:
curl -L -R -O https://www.lua.org/ftp/lua-5.4.7.tar.gz
tar zxf lua-5.4.7.tar.gz
cd lua-5.4.7
make all test
Check out Lua's FAQ page for more details.
Lua Language Syntax and Conventions
Let's now learn the Lua programming language basics with a brief overview of Lua's style, syntax, and typical conventions.
Lua Naming Conventions
According to Lua's own Lexical Conventions, the variables (called 'names' and 'identifiers') "can be any string of letters, digits, and underscores, not beginning with a digit", and 'camelCase' is the most typical convention used when declaring identifiers.
Naming Conventions for Lua Functions
As per their own documentation: "A function name consisting of multiple words may run together as in (getmetatable), as done in the standard Lua functions, though you may choose to use underscores (print_table)", and that some may also use "CapitalCase" (like MyFunction).
The naming conventions in Lua can feel a bit vague, as they often depend on the context and the specific practices of the Lua community. Here's a breakdown of the conventions mentioned in the documentation and some guidelines to help you decide:
Lua Conventions When Naming Variables
Local variables typically use snake_case or simple lowercase names.
- Short and Descriptive: For local variables, short and lowercase names (like color, count) are commonly used. It's acceptable to use single-letter names (like i) for loop counters or short-lived variables.
- Descriptive Globals: Global variables should have more descriptive names to avoid conflicts and improve readability. Avoid single-letter names for globals.
Lua Conventions When Naming Functions
- Underscores: Functions can be named using underscores to separate words (e.g., print_table).
- CamelCase: Some developers use CamelCase (e.g., GetValue), but this is less common in Lua's standard library.
- Consistency: Choose one style and stick to it within your project for consistency.
Other Lua Naming Conventions
- Prefix with is_ or has_: For Boolean values or functions that return a Boolean, it's helpful to prefix them (e.g., is_directory, has_permission). This improves readability by indicating the nature of the variable.
- Screaming Snake Case Constants: Constants should be named in uppercase with underscores separating words (e.g., MAX_LINES, PI_VALUE).
- Concatenated Words: Modules are typically named in lowercase with no separators (e.g., luasql, socket).
The main thing to keep in mind is being consistent in how you name things throughout the entire Lua application.
Lua Indentation
According to Lua's manual, its syntax and naming conventions are very similar to Python's. Lua uses indentation, however, unlike Python, it does not enforce indentation as part of its syntax, and is completely optional, although it's highly recommended to follow their indentation convention.
Here are some key points regarding indentation in Lua:
- Flexible Indentation: Lua does not require specific indentation levels to interpret blocks of code. You can use spaces or tabs, and you can indent code as much or as little as you like without causing syntax errors.
- Convention Over Enforcement: While Lua doesn't enforce indentation, it's a common convention to use consistent indentation for readability. Developers often use indentation to visually delineate code blocks, making it easier to understand the structure of the code.
- Brackets and Keywords: In Lua, code blocks are typically defined using keywords (like do, then, end) rather than indentation.
For example:
if condition then
-- This is indented for readability
print("Condition met")
end
In the above example, the print statement is indented to indicate that it belongs to the if block, but if you choose not to indent it, the code would still function correctly:
if condition then print("Condition met") end
Even though you could do something like the above, and write one-liners, you should ideally stick to Lua's indentation conventions whenever possible.
Learn About the Lua Basics
Here a some of the core functions, features, and practices to keep in mind while writing Lua scripts.
Lua's Print Function
To print output to the console, use the print function:
print("Hello, Lua!")
Lua Print Table Examples
To print a Lua table you'll have to use a for loop, like so:
mytable = {'hello', 'world'}
for k, v in pairs(mytable) do
print(k, v)
end
--[[ Prints
1 hello
2 world
--]]
The above example loops over the key-value pairs of the Lua table, and prints each one respectively.
Custom Lua Print Table Function
Another clever solution is to create your own custom, recursive function to handle this for you, like in the following example:
function tableprint(t)
if type(t) == 'table' then
local s = '{ '
for k, v in pairs(t) do
if type(k) ~= 'number' then
k = '"' .. k .. '"'
end
s = s .. '[' .. k .. '] = ' .. tableprint(v) .. ', '
end
return s .. '} '
else
return tostring(t) -- Use 't' instead of 'o'
end
end
You can then just pass the table to the tableprint() function call like so:
tableprint(mytable)
-- Prints: `{ [1] = hello, [2] = world, }`
Lua Variables and Data Types
Lua has several basic data types, including:
- Nil: Represents a non-existent value.
- Boolean: true or false.
- Number: Represents numeric values. Lua uses double-precision floating-point numbers.
- String: Textual data enclosed in double quotes " " or single quotes ' '.
- Table: The primary data structure in Lua, used for arrays, dictionaries, and more.
To declare variables you can either declare it like you would a Python variable, or use the local keyword:
local number = 42
local name = "Lua"
is_active = true
NOTE: Make sure to use the local keyword to declare variables whenever possible. If you don't do this it could potentially pollute the namespace with global declarations, and cause memory issues among other problems.
Basic Lua Operators
-Arithmetic Operators: +, -, *, /, % (modulo), ^ (exponentiation)
-Comparison Operators: ==, ~= (not equal), <, >, <=, >=
-Logical Operators: and, or, not
Lua Colon Operator
The Lua colon operator (:) is just syntactic sugar that simplifies method calls on tables, particularly when dealing with object-oriented programming concepts.
Even though Lua doesn't really support OOP, it nonetheless acts as a highly flexible and extensible language that allows developers to implement OOP concepts using its existing features, such as tables and metatables.
The following is an example of how to use it:
local Dog = {}
Dog.__index = Dog
function Dog:new(name)
local instance = setmetatable({}, Dog)
instance.name = name
return instance
end
function Dog:bark()
print(self.name .. " says Woof!")
end
local myDog = Dog:new("Buddy")
myDog:bark() -- Output: Buddy says Woof!
In this example:
- The new method creates a new instance of the Dog table and sets its name property.
- The bark method is defined with the colon operator, allowing it to access self.name.
Lua Dot Operator
When you define a function that doesn't require the self argument, you can use the dot operator (.) instead of the colon operator.
Example:
function Dog.sit()
print("Sitting...")
end
Dog.sit() -- This is called without an instance
Lua 'if' Statement with Multiple Conditions
Here's an example of how an if-else statement works in Lua:
local x = 10
if x > 5 then
print("x is greater than 5")
elseif x == 5 then
print("x is equal to 5")
else
print("x is less than 5")
end
Lua For Loop
A typical for loop in Lua works like this:
-- Numeric for loop
for i = 1, 5 do
print(i)
end
-- Generic for loop with iterators
local animals = {"cat", "dog", "bird"}
for index, value in ipairs(animals) do
print(index, value)
end
Lua 'for break' Example
Additionally, you can break out of these loops using the break statement. Here's a breakdown of the various loop types and how to break from them:
for i = 1, 10 do
print(i) -- Prints numbers from 1 to 10
if i == 5 then
break -- Breaks out of the loop when i equals 5
end
end
Here's another example that breaks out of a for loop in Lua:
local fruits = {"apple", "banana", "cherry"}
for index, value in ipairs(fruits) do
print(index, value)
if value == "banana" then
break -- Breaks out of the loop when the value is "banana"
end
end
Lua While Loop
A while loop looks like this:
local count = 1
while count <= 5 do
print(count)
count = count + 1
end
Lua 'repeat until' Example
The repeat loop in Lua is similar to a while loop, except you use it in conjunction with the until keyword to break out from it:
local count = 1
repeat
print(count)
count = count + 1
until count > 5
Lua Functions
Define functions using the function keyword in Lua:
function greet(name)
return "Hello, " .. name
end
print(greet("Lua"))
Lua Tables
Tables are the core data structure in Lua, used for arrays, dictionaries, and objects.
Creating a Table:
local person = {
name = "Alice",
age = 30,
is_employee = true
}
Accessing Table Elements:
print(person.name) -- Output: Alice
print(person["age"]) -- Output: 30
Using Tables as Arrays:
local colors = {"red", "green", "blue"}
print(colors[1]) -- Output: red (Lua arrays are 1-indexed)
Iterate Over Lua Tables
The ipairs() function in Lua is specifically designed for iterating over array-like tables (also known as sequences) that have integer keys starting from 1. It is a useful function for traversing tables where the elements are indexed by consecutive integers, making it straightforward to access each element in order. Here's a detailed overview of how ipairs() works:
Iterating Over Tables:
-- For array-like tables
for index, value in ipairs(colors) do
print(index, value)
end
-- For dictionary-like tables
for key, value in pairs(person) do
print(key, value)
end
Sequential Access: ipairs() iterates over the elements of the table starting from index 1 and continues until it encounters the first nil value.
This means that if the table has non-integer keys or if there are gaps in the integer sequence, ipairs() will stop iterating at the first gap.
Lua Table of Tables Example
Lua does not have built-in array or list types as found in many other programming languages. Instead, Lua uses tables as its primary and versatile data structure, which can be used to represent arrays, dictionaries (key-value pairs), and even more complex data structures.
Here's a simple example of a table containing other tables (also known as a nested table in Lua). This example represents a collection of students, where each student has their own details stored in a sub-table:
-- Table of students
local students = {
{ name = "Alice", age = 20, major = "Computer Science" },
{ name = "Bob", age = 22, major = "Mathematics" },
{ name = "Charlie", age = 21, major = "Physics" }
}
-- Iterate over the table of students and print their details
for _, student in ipairs(students) do
print("Name: " .. student.name)
print("Age: " .. student.age)
print("Major: " .. student.major)
print() -- Print a blank line for better readability
end
Notice how it uses the dot notation and ipairs() function to loop over and print the nested values.
Lua Remove From Table Examples
Removing an item from a Lua table can be done in several ways, depending on whether you're dealing with an array-like table (sequential indices) or a dictionary-like table (key-value pairs).
When dealing with an array (where indices are numeric), you can remove an item by setting its index to nil:
local fruits = {"apple", "banana", "cherry", "date"}
-- Remove "banana"
fruits[2] = nil
-- The table now has a nil value at index 2
for i, fruit in ipairs(fruits) do
print(i, fruit) -- This will skip the nil value
end
NOTE: Setting an item to nil in a Lua table can affect its length. Specifically, functions like # (the length operator) do not count nil values. As a result, if there are any nil entries, ipairs will skip over them, and you may not iterate over the entire table as expected. Consider using table.remove() to maintain the integrity of the array indices.
Using 'table.remove' in Lua
The table.remove function is useful for removing an element from an array-like table by shifting the subsequent elements down:
local fruits = {"apple", "banana", "cherry", "date"}
-- Remove the second element ("banana")
table.remove(fruits, 2)
-- The table now contains "apple", "cherry", and "date"
for i, fruit in ipairs(fruits) do
print(i, fruit) -- Outputs: 1 apple, 2 cherry, 3 date
end
Removing a Key-Value Pair from a Lua Table
To remove a key-value pair from a table that acts like a dictionary, simply set a key's value to nil:
local person = {
name = "Alice",
age = 30,
job = "Engineer"
}
-- Remove the "age" key
person.age = nil
-- The table now has the key "age" removed
for key, value in pairs(person) do
print(key, value) -- Outputs: name Alice, job Engineer
end
In Lua, you can remove items from tables in various ways, including:
- Setting an index to nil for array-like tables.
- Using table.remove() to shift elements in an array.
- Setting a key to nil to remove key-value pairs in dictionary-like tables.
- Filtering through a loop to create a new table with only the desired items.
Lua Type Checking
To check the type of a variable, use the type function:
print(type(number)) -- Output: number
print(type(name)) -- Output: string
print(type(person)) -- Output: table
print(type(is_active)) -- Output: boolean
Lua Metatables and Metamethods
Metatables allow you to define behavior for operations on tables (e.g., addition, indexing). For example:
local mt = {
__add = function(t1, t2)
return t1.value + t2.value
end
}
local a = {value = 10}
local b = {value = 20}
setmetatable(a, mt)
setmetatable(b, mt)
print(a + b) -- Output: 30
Lua ipairs vs pairs
Here's how ipairs() compares with the pairs() function:
- ipairs() is used for ordered, sequential arrays (integer keys starting from 1), and returns two values on each iteration (index and key).
- pairs() can be used for any table and iterates over all key-value pairs, regardless of their type, but does not guarantee order.
String Manipulation in Lua
Lua has built-in support for string manipulation, but its capabilities for regular expressions (regex) are somewhat limited compared to languages like Python or JavaScript. Here's an overview of how Lua handles string manipulation and pattern matching:
Let's now go over how Lua handles string manipulation and pattern matching.
Built-In Lua Functions for String Manipulation
Lua provides a set of built-in functions for string manipulation, such as the .. operator:
local str1 = "Hello"
local str2 = "World"
local result = str1 .. " " .. str2 -- "Hello World"
Use the # operator to get the length of a string.
local length = #result
-- length should now equal `11`
Use the string.sub function to extract substrings in Lua:
local substring = string.sub(result, 1, 5) -- "Hello"
Lua provides the string.gsub function for global substitution, and string.find for finding patterns:
local new_str = string.gsub(result, "World", "Lua") -- "Hello Lua"
RegEx Pattern Matching in Lua
Lua has a unique pattern matching system that is simpler than full regex, yet powerful for many use cases, and supports basic pattern syntax, such as:
- %a: Matches any letter.
- %d: Matches any digit.
- .: Matches any character except a newline.
- %s: Matches any space character.
- *, +, -, and ? can be used for repetition.
Let's go over some of the pattern functions in Lua you can use.
The string.match function returns the first match of a pattern in a string:
local match = string.match("Hello Lua", "%a+")
-- "Hello"
The string.gmatch: function returns an iterator for all matches of a pattern in a string:
for word in string.gmatch("Hello Lua", "%a+") do
print(word)
-- Prints "Hello" and "Lua"
end
The best way to search a string is to use string.find and look for a pattern which will return the starting and ending indices:
local start_pos, end_pos = string.find("Hello Lua", "Lua")
-- 7, 9
Lua's pattern matching is not as powerful or feature-rich as full regular expressions. It lacks certain constructs such as lookaheads, lookbehinds, and more complex grouping capabilities.
For users needing more advanced regex capabilities, there are third-party libraries available, such as Lrexlib (A binding to the PCRE), which allows Lua to use more advanced regex features.
Lrexlib can be installed with the LuaRocks package manager like so:
luarocks install lrexlib-FLAVOUR
Replace FLAVOUR with PCRE, PCRE2, POSIX, oniguruma, TRE, or GNU.
Error Handling in Lua
Error handling in Lua is primarily managed through a combination of the pcall() (protected call) and xpcall() functions, along with the use of error handling mechanisms.
The pcall() function allows you to call a function in protected mode. If the function executes successfully, it returns true followed by the function's return values. If it throws an error, it returns false followed by the error message:
function riskyFunction()
error("Something went wrong!")
end
local status, err = pcall(riskyFunction)
if not status then
print("Error:", err) -- Output: Error: Something went wrong!
end
Similar to pcall(), there is also a xpcall() function that allows you to specify an error handler function that will be called in case of an error. This is useful for custom error handling logic:
function errorHandler(err)
return "Handled error: " .. err
end
local status, err = xpcall(riskyFunction, errorHandler)
if not status then
print(err) -- Output: Handled error: Something went wrong!
end
Throwing Errors in Lua
You can throw errors using the error() function, which can be used to signal that something went wrong in your code:
function divide(a, b)
if b == 0 then
error("Division by zero")
end
return a / b
end
Best Practices for Error Handling
- Use pcall() for Safe Function Calls: Always wrap potentially error-throwing function calls in pcall() or xpcall() to gracefully handle errors without crashing the program.
- Custom Error Handlers: If you have specific error handling logic, use xpcall() to define how to manage different types of errors more effectively.
- Return Error Information: When defining functions, consider returning error information (like nil and an error message) rather than using error() to allow the caller to decide how to handle the situation.
- Keep Error Messages Clear: Ensure that error messages are informative to help with debugging. Include relevant context about what caused the error.
- Graceful Degradation: Implement fallback logic or default values to ensure that your application can continue running even if certain operations fail.
Here's an example of a function returning an error message (similar to how Go safely handles errors):
function safeDivide(a, b)
if b == 0 then
return nil, "Division by zero"
end
return a / b
end
local result, err = safeDivide(10, 0)
if not result then
print("Error:", err) -- Output: Error: Division by zero
end
Debugging Lua Errors
Use debug.traceback() in conjunction with error handling to get a stack trace when an error occurs, which can help identify where the error originated:
function riskyFunction()
error("Something went wrong!")
end
local function callWithTrace()
return riskyFunction()
end
local status, err = xpcall(callWithTrace, function(err)
return debug.traceback(err)
end)
if not status then
print(err) -- Outputs the error with a stack trace
end
Lua vs Python
One significant advantage Lua holds over Python is its speed—Lua is designed to be fast and lightweight, often outperforming Python in execution time, especially for embedded systems and game development. Lua's close integration with C allows for seamless C bindings, giving it an edge in performance-critical applications where direct interaction with system-level functions is necessary. While Python excels in a broad range of applications with an extensive standard library, Lua shines when high performance, simplicity, and small footprint are priorities.
Lua Tables vs Python Dictionaries
Lua tables and Python dictionaries are both versatile data structures used for storing collections of data, but they have some key differences in design, functionality, and usage. Here's a comparison of the two:
- A table in Lua is a general-purpose data structure that can be used as an array, a dictionary (key-value pairs), or even an object (using metatables).
- It can hold values of any type, including other tables, making it extremely flexible.
In Lua, keys can be any Lua type, including numbers, strings, and even other tables. However, tables can only use strings and numbers as keys in practice.
Python Dictionaries
A dictionary in Python is specifically designed to store key-value pairs. A Python dictionary also allows keys of various immutable types (like strings, numbers, or tuples) and values of any type, but it is primarily focused on the mapping of keys to values.
Python vs Lua Array Behavior
Lua Table:
- Tables can function as arrays, allowing for numerical indexing starting from 1 (Lua uses 1-based indexing).
- You can use integer keys for array-like access, allowing for a mix of array and dictionary behavior.
Python Dictionary:
- Dictionaries do not support numerical indexing in the same way arrays or lists do. They are strictly key-value pairs and do not maintain order until Python 3.7, when insertion order was guaranteed.
Lua For Loop
-Lua Table:
- You typically use the pairs() function to iterate over key-value pairs and ipairs() for iterating over array-like sequences (1-based).
local myTable = {a = 1, b = 2}
for k, v in pairs(myTable) do
print(k, v)
end
Loop Dict in Python
You can iterate over keys, values, or items directly using a for loop.
my_dict = {'a': 1, 'b': 2}
for k, v in my_dict.items():
print(k, v)
Lua Performance vs Python
Both data structures are implemented to provide efficient lookups, insertions, and deletions, but performance can vary depending on specific use cases and implementation.
Metatables (Lua Specific):
- Lua Table: Lua allows you to define metatables, enabling you to change the behavior of tables, such as how they handle indexing or arithmetic operations.
- Python Dictionary: Python dictionaries do not have a built-in mechanism for metaprogramming like Lua's metatables, although you can subclass the dict class to customize behavior.
In summary, while Lua tables and Python dictionaries serve similar roles in storing collections of data, they differ in structure, behavior, and capabilities. Lua tables are more flexible, supporting multiple data types and serving multiple roles (arrays, dictionaries, objects), while Python dictionaries are specifically focused on key-value pair storage with immutable keys. Each has its strengths, depending on the programming context and use case.
Lua Embedded in Multiple Projects
Lua is widely used as an embedded scripting language in various applications and platforms beyond Neovim and Roblox. Here are some notable examples, whiuch neovim being .
1. Game Development:
- Roblox: Roblox uses Lua for scripting games within its platform, allowing users to create interactive experiences and game logic.
- Corona SDK: This cross-platform framework for mobile app development uses Lua for scripting games and apps, enabling developers to write code once and deploy on multiple platforms.
- Love2D: Love2D is a popular 2D game engine that uses Lua for game development, providing a simple and efficient framework for creating games.
2. Embedded Systems:
- OpenResty: A web platform that embeds Lua into Nginx, allowing developers to write web applications with Lua for handling requests and responses efficiently.
- ESP8266/ESP32: These popular microcontrollers can run Lua scripts using the NodeMCU firmware, making it easier to develop Internet of Things (IoT) applications.
3. Software Applications:
- Wireshark: The network protocol analyzer uses Lua for writing custom dissectors and plugins, allowing users to extend its functionality.
- Adobe Lightroom: Uses Lua for scripting to automate tasks and customize workflows within the application.
4. Simulation and Modeling:
- Dwarf Fortress: Lua is used for scripting mods and extensions in this popular simulation game, allowing users to create custom features and behaviors.
- Factorio: While the primary scripting language is Lua, players can write scripts to enhance gameplay, create mods, and automate processes.
5. Scripting in Various Applications:
- World of Warcraft: Lua is used for creating add-ons, allowing players to customize their interface and gameplay experience.
- The Sims Series: Some versions allow scripting with Lua for creating custom game mechanics and interactions.
6. Networking and Server Management:
- OpenWrt: This Linux distribution for embedded devices allows scripting with Lua for managing router configurations and automating tasks.
- Lighttpd: This web server can be extended with Lua to handle custom request processing and dynamic content generation.
7. IDEs:
- Neovim: The popular IDE nvim also embeds Lua to provide a powerful and flexible scripting interface for configuration, plugins, and extensions.
Lua's versatility and lightweight nature make it an excellent choice for embedding in a variety of applications, particularly in gaming, web development, embedded systems, and software applications. Its ability to provide a powerful yet easy-to-use scripting environment has contributed to its popularity across different domains.
Lua Drawbacks and Limitations
While Lua is a powerful and efficient language, especially for embedded systems and game development, it does have some notable limitations compared to languages like Python or Node.js, particularly in terms of libraries and built-in features. Here are some key areas where Lua may fall short:
1. Scientific Computing and Data Analysis:
- Missing Libraries: Lua lacks libraries equivalent to Python's NumPy and Pandas, which provide extensive capabilities for numerical computations and data manipulation.
- While there are some libraries for numerical operations in Lua (like Torch, primarily used for machine learning), they do not match the breadth and depth of Python's offerings.
2. Machine Learning and AI:
- Limited ML Libraries: While there are some machine learning libraries in Lua, such as Torch (now transitioned to PyTorch in Python), it lacks the comprehensive ecosystem found in Python with libraries like TensorFlow, Keras, and Scikit-learn.
3. Web Development Frameworks:
- Fewer Options: Lua has web frameworks like Lapis and Sailor, but these are less popular and feature-rich compared to Python's Django and Flask or Node.js frameworks like Express. The community support and ecosystem around Lua for web development are not as robust.
4. Data Science and Visualization:
- Visualization Libraries: Lua has very few libraries for data visualization, making it less suitable for data science projects compared to Python's Matplotlib, Seaborn, or Plotly.
5. Concurrency and Asynchronous Programming:
- Limited Support: While Lua provides coroutines for cooperative multitasking, it lacks built-in support for asynchronous programming like Node.js's event-driven model, which is particularly useful for I/O-bound tasks.
6. Standard Library Features:
- Less Comprehensive Standard Library: Lua's standard library is more minimalistic compared to Python's extensive standard library, which includes modules for everything from file I/O to regular expressions and internet protocols.
7. Object-Oriented Programming (OOP):
- No Built-in OOP Support: Lua does support OOP through its metatables, but it doesn't have built-in OOP features like Python's classes and inheritance. This can make designing complex systems more cumbersome.
8. Community and Ecosystem:
- Smaller Community: Lua has a smaller community compared to Python and Node.js, resulting in fewer available libraries, resources, and tutorials. This can be a significant barrier for new developers looking for help.
While Lua is highly efficient and excellent for specific use cases (especially in game development and embedded systems), it lacks many of the libraries and features that make Python and Node.js appealing for scientific computing, machine learning, web development, and general-purpose programming. If your project requires extensive libraries for data manipulation, machine learning, or web frameworks, Python or Node.js may be better suited for your needs.
Conclusion
As we've explored in this article, Lua offers a unique blend of simplicity, flexibility, and performance, making it an excellent choice for applications ranging from game development to embedded systems. Its lightweight nature and ease of embedding within other applications allow developers to extend functionalities and add scripting capabilities without significant overhead. Lua's syntactical simplicity contributes to a short learning curve, further aiding its adoption across various sectors.
However, while Lua excels in performance and integration, it does have limitations, especially when compared to languages like Python, in terms of available libraries and community support for certain applications like web development and data science. These limitations underscore the importance of choosing the right tool for the job based on the specific needs and constraints of your project.
In conclusion, Lua is not just a powerful scripting language but also a versatile tool in a developer's arsenal, well-suited for those who need a fast, efficient, and easily integrable language with a gentle learning curve. Whether you're developing a mobile game, scripting for a high-performance web server, or creating custom features in a software application, Lua's capabilities should be strongly considered alongside more popular languages like Python and JavaScript.