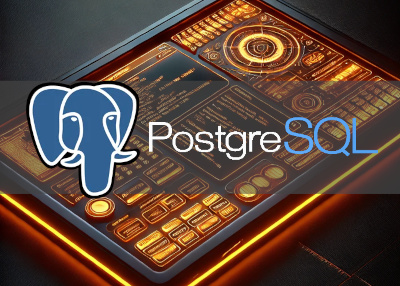
Working with Timestamps and Dates in PostgreSQL
PostgreSQL provides great support for handling timestamps and dates, making it a powerful tool for managing temporal data. Whether you're dealing with simple date values or complex timestamp operations, PostgreSQL offers a range of features to meet the needs of any project.
DISCLAIMER: The bulk of this article was written by ChatGPT v4o with some modifications applied by a human being. Use this information with caution and discrection.
Understanding PostgreSQL Timestamps and Dates
In PostgreSQL, timestamps and dates are managed using the following data types:
- timestamp without time zone: Stores date and time without timezone information. It represents a point in time, but does not account for timezone differences.
- timestamp with time zone: Stores date and time with timezone information. This is the preferred type for applications that require precise timekeeping across different timezones.
- date: Stores only the date part (year, month, day) without time.
- time without time zone: Stores only the time part (hours, minutes, seconds) without date and timezone.
- time with time zone: Stores time with timezone information.
Setting Timezone Defaults
When working with timestamps, it's important to consider timezone handling to ensure consistency across different regions. PostgreSQL allows you to set the timezone at the session level or for the entire database. For example:
SET timezone='UTC';
This command sets the timezone for the current session to UTC. However, this setting will only affect the current session and does not persist across connections.
Enforcing UTC Timezone in Table Columns
To ensure that timestamps are consistently stored in UTC, you can define custom functions to return the current UTC timestamp. PostgreSQL does not allow expressions like CURRENT_TIMESTAMP AT TIME ZONE 'UTC' to be used directly as default values for columns. Instead, you can create a custom function to handle this requirement.
Create a Custom Function
The next step is to create a function that returns the current timestamp in UTC. Here's how to define, and use, a custom function to enforce UTC timestamps:
CREATE OR REPLACE FUNCTION utc_now()
RETURNS timestamp with time zone AS $$
BEGIN
RETURN CURRENT_TIMESTAMP AT TIME ZONE 'UTC';
END;
$$ LANGUAGE plpgsql;
This function utc_now() will always return the current time in UTC, which can be used as a default value for timestamp columns.
Using the Custom Function in Table Definitions
When defining your table schema, you can use this custom utc_now() function as a default value for timestamp columns:
CREATE TABLE example_table (
id SERIAL PRIMARY KEY,
created_at TIMESTAMP WITH TIME ZONE DEFAULT utc_now(),
updated_at TIMESTAMP WITH TIME ZONE DEFAULT utc_now()
);
This ensures that whenever a row is inserted without specifying a value for created_at or updated_at, the current UTC timestamp is automatically assigned.
Querying Date Ranges in PostgreSQL
At some point, while using PostgreSQL, you will need to query date ranges, and filter records within a specific period—perhaps even compare timestamps. PostgreSQL provides powerful querying capabilities to handle these requirements efficiently.
Basic Date Range Queries
To query records within a specific date range, you can use the BETWEEN operator or comparison operators. Here's a basic example using the BETWEEN operator:
SELECT *
FROM your_table
WHERE your_date_column BETWEEN '2024-01-01' AND '2024-12-31';
This query retrieves all records where your_date_column falls within the year 2024.
Using Comparison Operators in psql
You can also use comparison operators for more flexibility and precision:
SELECT *
FROM your_table
WHERE your_date_column >= '2024-01-01'
AND your_date_column < '2025-01-01';
The above command should retrieve records from January 1, 2024, to December 31, 2024, but it will also exclude January 1, 2025.
Handling Postgres Timestamps
When dealing with timestamps, you may want to include or exclude specific times of the day. For example, to find records created on a specific day but within any time of that day:
SELECT *
FROM your_table
WHERE your_timestamp_column::date='2024-08-15';
This query converts your_timestamp_column to a date format, effectively ignoring the time component, and retrieves all records for August 15, 2024.
Interval-Based psql Queries
To query records based on intervals, you can use PostgreSQL's INTERVAL type:
SELECT *
FROM your_table
WHERE your_timestamp_column >= NOW() - INTERVAL '30 days';
This query retrieves all records from the past 30 days based on the current timestamp.
Combining Date and Time Functions
You can combine date and time functions for more advanced queries. For instance, to find records from the start of the current month to the end of the current month:
SELECT *
FROM your_table
WHERE your_timestamp_column >= DATE_TRUNC('month', NOW())
AND your_timestamp_column < DATE_TRUNC('month', NOW()) + INTERVAL '1 month';
This query should fetch for you all the records, from the beginning of the current month to the end, but it will also exclude the next month's records.
Conclusion
This should give you a broad overview of PostgreSQL's timestamp and date handling features, along with custom functions for UTC enforcement, so that you can ensure consistent and accurate timekeeping across your application. Properly managing timestamps and dates is crucial for applications that operate across multiple timezones or require precise temporal data.