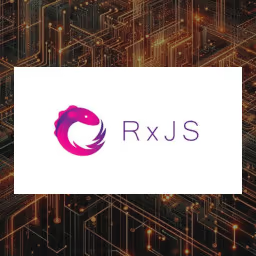
RxJS Observables and Operators
In the ever-evolving landscape of modern web development, managing asynchronous data flows and complex event-driven interactions is a critical challenge. This is where RxJS (Reactive Extensions for JavaScript) steps in—a powerful library designed to handle streams of data in a flexible, composable, and reactive way. At the heart of RxJS lies the concept of observables, which allow developers to work with everything from HTTP requests to user input events as continuous data streams. Unlike traditional promises or callbacks, observables empower developers to elegantly combine, manipulate, and transform data in real-time. This article dives into the fundamentals of RxJS and observables, explores why they've become a cornerstone of frameworks like Angular, and highlights their typical use cases, such as handling user interactions, real-time updates, state management, and complex asynchronous workflows. Whether you're building live dashboards, search functionality, or scalable APIs, RxJS equips you with the tools to do so with clarity and control.
What is an Observable, and How Does It Differ from Promises?
Think of an RxJS observable as a "blueprint" for producing a stream of data over time. Observables allow you to handle events or asynchronous data in a powerful, composable way. Here's how they differ from promises and why they're special.
Key Characteristics of Observables
Lazy execution:
Observables don't do anything until someone subscribes to them:
- You can think of it like a function that doesn't run until it's called.
- Promises, by contrast, start executing immediately once created.
Example:
const observable = new Observable((observer) => {
console.log("Observable logic running");
observer.next("Hello");
});
// Nothing happens yet—no subscription
console.log("Before subscription");
observable.subscribe((data) => console.log(data)); // Now it executes
Multiple emissions
Observables can emit multiple values over time (like a stream of data), whereas promises resolve only once.
Example:
const observable = new Observable((observer) => {
observer.next(1);
observer.next(2);
observer.next(3);
observer.complete(); // No more emissions after this
});
observable.subscribe((value) => console.log(value));
// Output: 1, 2, 3
Cancellable
You can "unsubscribe" from an observable to stop receiving data. With promises, there's no built-in cancellation mechanism once the operation starts.
Example:
const subscription = observable.subscribe((value) => console.log(value));
subscription.unsubscribe(); // Stops further emissions
Powerful RxJS operators
Observables have RxJS operators (map, filter, switchMap, etc.) that let you transform, combine, and manipulate data streams easily.
How Observables Compare to Promises
Feature | Promise | Observable |
---|---|---|
Execution | Eager (starts immediately) | Lazy (starts only on subscription) |
Emissions | Single value | Multiple values over time |
Cancellation | Not directly cancellable | Can unsubscribe to stop emissions |
Composition | Limited chaining (.then) | Rich set of operators |
Why Observables Are Useful in Angular
Angular uses observables heavily for handling asynchronous tasks like:
1. HTTP requests (HttpClient returns observables).
2. Event streams (e.g., DOM events, user input).
3. Reactive forms (value and status changes are observables).
Observables are especially helpful because they:
- Let you handle multiple events over time.
- Can be canceled if a component is destroyed (avoiding memory leaks).
- Have operators to simplify complex asynchronous logic, like with the switchMap operator.
Summary
1. Error Handling: Errors in the pipe chain bubble down to the final subscribe.error by default. Use catchError to handle specific errors in the chain.
2. Observable Basics:
- Observables are lazy, can emit multiple values, and are cancelable.
- They provide a more flexible, composable way to handle async operations compared to promises.
3. Why RxJS in Angular: Observables integrate seamlessly with Angular's reactive programming model, making them perfect for tasks like HTTP requests and event handling.
How is RxJS Used?
Let's explore the relationship between RxJS and modern JavaScript frameworks/libraries, focusing on their levels of support and usage.
1. RxJS Beyond Angular
RxJS is not exclusive to Angular—it's a standalone library that can be used with any JavaScript application, regardless of the framework. However, Angular tightly integrates RxJS into its ecosystem, making it a core part of how Angular developers handle asynchronous data.
Why Angular Loves RxJS
- Angular's HttpClient is based on observables, so all HTTP requests return RxJS observables.
- Forms, routing, and event handling in Angular can all use RxJS observables to create reactive, composable workflows.
- Angular's core architecture (e.g., dependency injection, change detection) aligns well with the reactive programming paradigm RxJS promotes.
Other frameworks or libraries (like React, Vue, or Svelte) don't require RxJS, but it can still be used in these environments. Let's look at how well it's supported.
2. RxJS in Other Frameworks
React
React doesn't natively use or depend on RxJS, but you can easily incorporate it. For example:
- State Management: Libraries like RxJS for state let you manage global or local state in a reactive way, similar to Redux but with RxJS operators.
- Event Streams: React's event handling can benefit from RxJS when you need to process or combine multiple streams of data (e.g., user inputs, web socket streams).
- Custom Hooks: You can use RxJS to create powerful, reusable hooks that integrate with React's lifecycle.
Example of an RxJS-powered custom hook:
import { useEffect, useState } from "react";
import { Subject } from "rxjs";
import { debounceTime, distinctUntilChanged, switchMap } from "rxjs/operators";
export const useSearch = (searchFn) => {
const [results, setResults] = useState([]);
const searchSubject = new Subject<string>();
useEffect(() => {
const subscription = searchSubject
.pipe(
debounceTime(300),
distinctUntilChanged(),
switchMap((query) => searchFn(query))
)
.subscribe(setResults);
return () => subscription.unsubscribe(); // Cleanup on unmount
}, [searchFn]);
return { search: (query) => searchSubject.next(query), results };
};
Vue
Vue doesn't natively depend on RxJS either but integrates well with it:
- Libraries like RxJS-Vue and RxVue make it simple to use observables in Vue components.
- Vue's reactive system can feel similar to RxJS in some ways, but RxJS brings more advanced capabilities (e.g., stream combination).
Svelte
Svelte has its own reactive system built into the framework, so RxJS isn't as commonly used. However:
- You can still integrate RxJS for use cases like web socket streams, complex async workflows, or state management.
- Svelte stores can work alongside RxJS observables for added flexibility.
Node.js
In Node.js, RxJS can be used for:
- File streams (processing logs, handling streaming APIs).
- Event-driven applications (e.g., chaining event listeners with observables).
- WebSocket-based communication for real-time data streams.
Example: Reading a file stream with RxJS:
import { fromEvent } from "rxjs";
import { map, takeUntil } from "rxjs/operators";
import * as fs from "fs";
const stream = fs.createReadStream("largefile.txt");
const data$ = fromEvent(stream, "data");
const end$ = fromEvent(stream, "end");
data$
.pipe(
map((chunk) => chunk.toString()),
takeUntil(end$) // Automatically complete when file reading ends
)
.subscribe({
next: (data) => console.log(data),
complete: () => console.log("File read complete."),
});
Angular Support for RxJS
Angular supports all RxJS features, as RxJS is fully integrated into Angular's ecosystem. However, the way Angular uses RxJS often emphasizes certain patterns and operators over others:
- Angular's core APIs (like HttpClient, forms, and router events) already use observables, so you don't need to "reinvent" how to use RxJS in most cases.
- Most Angular developers only use a subset of RxJS features because the framework abstracts many reactive complexities for you.
Examples of heavily used RxJS features in Angular:
- Operators like switchMap, mergeMap, catchError, map, debounceTime.
- Subject-based constructs (e.g., BehaviorSubject for state management).
However, advanced RxJS features (like multicasting or custom operators) are fully available in Angular but are not commonly needed for everyday Angular apps.
4. Why RxJS Isn't As Popular Outside Angular
RxJS's power lies in handling streams of data and complex async workflows, but many modern frameworks solve these problems differently:
- React uses useState, useEffect, and libraries like Redux or Zustand for state management, so developers don't need RxJS for common use cases.
- Vue has its built-in reactivity system.
- Svelte relies on its stores and lifecycle methods for reactive programming.
While RxJS is powerful, its learning curve and verbose syntax can make it overkill for simpler applications. For frameworks that don't deeply integrate RxJS (like Angular does), alternatives like promises or native async/await are often sufficient.
5. Summary
- RxJS is universal: It works with any JavaScript framework/library or even vanilla JS.
- Angular fully supports RxJS: Angular tightly integrates RxJS into its ecosystem, leveraging its full power for HTTP requests, event handling, forms, and routing.
- Other frameworks can use RxJS, but they don't depend on it as Angular does. Alternatives (like React's hooks or Svelte's stores) often fulfill similar needs with simpler syntax.
When to Use RxJS Outside Angular
- Real-time systems: WebSockets, live updates, or real-time event handling.
- Complex async workflows: Combining multiple streams, error handling, or managing multiple dependent tasks.
- Advanced state management: RxJS can replace Redux or similar libraries in React or Vue when you need more flexibility.
Conclusion
RxJS is like a toolbox for handling streams and asynchronous data—use it when the benefits outweigh the complexity! 😊