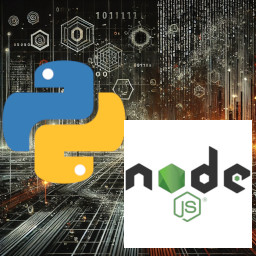
Code Secure Random Password Generators
Randomness is a fundamental aspect of programming, playing a crucial role in various applications such as simulations, games, cryptography, and security protocols. However, not all randomness is created equal. While standard random number generators (RNGs) are suitable for tasks like simulations and modeling, they fall short in security-sensitive applications. This is where cryptographically secure pseudorandom number generators (CSPRNGs) come into play.
In this article, we will delve into the differences between standard and cryptographically secure randomness. We'll explore how to generate random values using both methods in Python and JavaScript, highlighting their use cases, strengths, and limitations.
DISCLAIMER: The content of this article was generated from ChatGPT prompts, and curated/edited by a human being.
Comparing Standard and Cryptographic Randomness
Predictability
- Standard Randomness: Predictable if the seed or internal state is known.
- Cryptographic Randomness: Designed to be unpredictable, even if part of the output is known.
Security Considerations
- Standard Randomness: Not suitable for security-sensitive applications.
- Cryptographic Randomness: Essential for generating keys, tokens, and passwords securely.
Performance Considerations
- Standard Randomness: Generally faster due to simpler algorithms.
- Cryptographic Randomness: Slightly slower but acceptable for most applications where security is a priority.
Let's now explore how we can implement cryptographically secure random number generation in Python and Node.js. We'll discuss why it's essential to use secure methods, the risks associated with insecure randomness, and provide practical code examples to guide you.
Crypto Security and Randomness
Random number generation is a critical component in various applications, particularly those involving cryptography and security. In contexts like password generation, token creation, and encryption, the randomness must be unpredictable and resistant to attacks. This is where Cryptographically Secure Pseudorandom Number Generators (CSPRNGs) come into play.
Why Cryptographically Secure Randomness Matters
As covered earlier, standard random number generators, such as Python's random module or JavaScript's Math.random(), are not suitable for security-sensitive applications. They are deterministic and can be predictable if the internal state or seed is known, making them vulnerable to attacks.
Cryptographically secure random number generators are designed to be unpredictable and resistant to reverse-engineering. They gather entropy from various non-deterministic sources, making it computationally infeasible to predict future values even if part of the sequence is known.
Cryptographic libraries provide essential tools for generating secure random numbers, which are crucial in various applications, especially in security and cryptography. Here's an overview of cryptographic libraries for random analysis in Python and Node.js.
Why Standard Random Functions Are Not Suitable for Security Purposes
Standard RNGs are deterministic and can be predicted if the internal state or seed is known. This makes them vulnerable in security contexts where unpredictability is paramount, such as in generating encryption keys, tokens, or passwords.
Standard Randomness in Python and JavaScript
For both Python and Node.js, it's crucial to utilize dedicated cryptographic libraries when working with random number generation in security contexts. Libraries like secrets and os.urandom() in Python, along with the crypto module in Node.js, provide secure and efficient ways to generate random values that are essential for cryptographic applications, password management, and more. Always ensure you're using these tools appropriately to maintain the security of your applications.
It's essential to use these libraries, instead of less secure methods like random in Python or Math.random() in JavaScript, however, let's briefly go over the standard random libraries for each language.
Random Numbers in Python
Using Python's random module or NumPy's np.random library are among the most common ways to generate random numbers in Python.
Python's 'random' Module
The random module in Python is a widely used library for generating pseudorandom numbers. It is based on the Mersenne Twister algorithm, which is fast and has a very long period.
It should be emphasized that the random module is not suitable for cryptographic purposes. It is intended for modeling and simulation, not security.
Avoid doing this when security is a concern:
import random
# Generate a random float between 0.0 and 1.0
print(random.random())
# Generate a random integer between 1 and 10
print(random.randint(1, 10))
# Choose a random element from a list
options = ['apple', 'banana', 'cherry']
print(random.choice(options))
Characteristics of Python Random:
- Deterministic: Given the same initial seed, the sequence of numbers generated will be the same.
- Not Secure: The predictability makes it unsuitable for cryptographic purposes.
- Use Cases: Simulations, games, and statistical modeling.
Python NumPy Random Example
NumPy is a powerful library in Python primarily used for numerical computations. It includes a variety of functions for generating random numbers, which can be useful in various applications such as simulations, statistical analysis, and machine learning.
Here's a basic example that demonstrates how to use NumPy to generate random numbers:
import numpy as np
# Set a random seed for reproducibility
np.random.seed(42)
# Generate an array of 5 random floats between 0 and 1
random_floats = np.random.rand(5)
# Generate an array of 5 random integers between 1 and 100
random_integers = np.random.randint(1, 101, size=5)
print("Random Floats:", random_floats)
print("Random Integers:", random_integers)
1. Setting a Random Seed: Using np.random.seed(42) ensures that the random numbers generated are reproducible. This means that every time you run the code with the same seed value, you will get the same random numbers. This is important in scenarios like testing and debugging.
2. Generating Random Floats: The np.random.rand(5) function generates an array of 5 random floats uniformly distributed between 0 and 1.
3. Generating Random Integers: Then np.random.randint(1, 101, size=5) generates an array of 5 random integers, each between 1 and 100 (inclusive of 1 and exclusive of 101).
Why Use NumPy for Random Number Generation?
1. Simulations: In scientific research or financial modeling, random numbers are often used to simulate various scenarios (e.g., Monte Carlo simulations).
2. Statistical Analysis: Generating random samples is crucial for performing statistical analyses, hypothesis testing, or creating confidence intervals.
3. Machine Learning: Randomness plays a vital role in machine learning algorithms, particularly in initializing weights, shuffling data, and creating random subsets of data (e.g., for cross-validation).
4. Performance: NumPy's random number generation is highly optimized for performance, allowing for efficient operations on large arrays of random numbers, making it preferable for applications that require a large volume of random data.
NumPy is an excellent choice for random number generation in Python due to its efficiency and versatility. By using functions like np.random.rand() and np.random.randint(), you can easily generate random numbers for a variety of applications in data analysis, simulations, and machine learning, but, like random, it is not secure for cryptographic purposes.
Random Numbers in JavaScript
For pseudorandom number generation, in JavaScript or NodeJS, people typically use Math.random.
Math.random JavaScript Example
In JavaScript, the Math.random() function returns a pseudorandom number between 0 (inclusive) and 1 (exclusive).
// Generate a random float between 0.0 and 1.0
console.log(Math.random());
// Generate a random integer between 1 and 10
console.log(Math.floor(Math.random() * 10) + 1);
// Choose a random element from an array
const options = ["apple", "banana", "cherry"];
console.log(options[Math.floor(Math.random() * options.length)]);
Characteristics of Math.random:
- Not Secure: Like Python's random, it's not suitable for cryptographic applications.
- Implementation-Dependent: The algorithm may vary between different JavaScript engines.
- Use Cases: Non-security-critical applications like UI effects and simple games.
Cryptographic Random Number Generators (CSPRNG)
CSPRNGs are designed to be unpredictable and secure. They gather entropy from various non-deterministic sources, making it infeasible to predict future values even if part of the sequence is known.
Cryptographic Randomness in Python
Python provides several modules for generating cryptographically secure random values:
- Python Crypto Secure Random Using 'secrets': Introduced in Python 3.6, the secrets module is designed for generating secure tokens and passwords.
- Python's os.urandom Function: The os.urandom function returns a string of random bytes suitable for cryptographic use.
- Python's SystemRandom Class: As stated in the Python doc's, "[SystemRandom is] not available on all systems, does not rely on software state, and sequences are not reproducible".
Python Random Security or Secrets Example
The secrets module is specifically designed for generating cryptographically strong random numbers suitable for managing data such as passwords, account authentication, and security tokens.
The following is how you can use Python for random security or secrets:
import secrets
# Generate a secure random integer between 1 and 10
print(secrets.randbelow(10) + 1)
# Generate a secure random byte string
print(secrets.token_bytes(16))
# Generate a secure random URL-safe text string
print(secrets.token_urlsafe(16))
# Choose a secure random element from a list
options = ['apple', 'banana', 'cherry']
print(secrets.choice(options))
Python 'secrets' Example with 'token_hex'
Here's another example that generates secure random numbers in Python using token_hex():
import secrets
# Generate a secure random integer between 0 and 9
secure_int = secrets.randbelow(10)
print(f"Secure Random Integer: {secure_int}")
# Generate a secure random byte string
secure_bytes = secrets.token_bytes(16)
print(f"Secure Random Bytes: {secure_bytes}")
# Generate a secure random hexadecimal string
secure_hex = secrets.token_hex(16)
print(f"Secure Random Hex String: {secure_hex}")
# Generate a secure URL-safe text string
secure_url = secrets.token_urlsafe(16)
print(f"Secure Random URL-safe String: {secure_url}")
Python's Secure Random Generation Using Secrets
Here's another example of Python's secrets' library to implement secure password generation:
import secrets
import string
def generate_secure_password(length=12):
alphabet = string.ascii_letters + string.digits + string.punctuation
password = ''.join(secrets.choice(alphabet) for _ in range(length))
return password
secure_password = generate_secure_password()
print(f"Secure Password: {secure_password}")
Python Random Security 'urandom' Example:
Here the os.urandom function returns a bytes string called random_bytes:
import os
# Generate 16 random bytes
random_bytes = os.urandom(16)
print(random_bytes)
The os.urandom() function returns a string of random bytes suitable for cryptographic use.
Python SystemRandom Generator
The SystemRandom class uses os.urandom under the hood.
SystemRandom Python Example
import random
secure_random = random.SystemRandom()
# Generate a secure random integer between 1 and 10
print(secure_random.randint(1, 10))
# Choose a secure random element from a list
options = ['apple', 'banana', 'cherry']
print(secure_random.choice(options))
NodeJS Crypto and Randomness with JavaScript
In JavaScript, both in the browser and Node.js, cryptographic randomness can be achieved using built-in modules. Let's now go over some crypto libraries for random JavaScript generation and creating secure tokens.
Using 'crypto.getRandomValues()' (Browser)
The crypto.getRandomValues() method fills a typed array with cryptographically secure random numbers.
Crypto.getrandomvalues vs Math.random Example
Here's a simple crypto.getRandomValues() JavaSvript example that should be used in the browser only:
// Generate a secure random integer between 1 and 10
const array = new Uint32Array(1);
window.crypto.getRandomValues(array);
const randomInt = (array[0] % 10) + 1;
console.log(randomInt);
// Generate a secure random byte array
const byteArray = new Uint8Array(16);
window.crypto.getRandomValues(byteArray);
console.log(byteArray);
Notice how the getRandomValues() call requires a Uint8Array byte array be passed to it, instead of just a number like Math.random() calls do.
Using 'crypto.randomBytes()' (Node.js)
In Node.js, the crypto module provides cryptographic functionalities, including secure random number generation.
NodeJS 'randomBytes' Example
The crypto module's randomBytes() function generates cryptographically strong pseudo-random data:
const crypto = require("crypto");
// Generate 16 secure random bytes
crypto.randomBytes(16, (err, buffer) => {
if (err) throw err;
console.log(buffer);
});
// Generate a secure random integer between 1 and 10
function secureRandomInt(min, max) {
const range = max - min + 1;
if (range > 256) {
throw new Error("Range too large");
}
let randomNumber;
do {
randomNumber = crypto.randomBytes(1)[0];
} while (randomNumber >= 256 - (256 % range));
return min + (randomNumber % range);
}
console.log(secureRandomInt(1, 10));
Synchronous 'randomBytes' NodeJS Calls
If you don't want to use an asynchronous callback, then you can also write synchronous code for crypto.randomBytes() calls, like in the following example:
const crypto = require("crypto");
// Generate 16 secure random bytes synchronously
const secureBytes = crypto.randomBytes(16);
console.log("Secure Random Bytes:", secureBytes);
Generating Secure Random Integers
Node.js does not provide a direct method to generate secure random integers within a specific range, but you can create one using crypto.randomBytes().
JavaScript Secure Random Integer Example
const crypto = require("crypto");
function secureRandomInt(min, max) {
const range = max - min;
if (range <= 0) {
throw new Error("The max must be greater than the min.");
}
// Calculate the number of bytes needed to represent the range
const numBytes = Math.ceil(Math.log2(range) / 8);
const maxNum = Math.pow(256, numBytes);
let randomNumber;
do {
randomNumber = parseInt(crypto.randomBytes(numBytes).toString("hex"), 16);
} while (randomNumber >= maxNum - (maxNum % range));
return min + (randomNumber % range);
}
// Generate a secure random integer between 0 and 9
const secureInt = secureRandomInt(0, 10);
console.log("Secure Random Integer:", secureInt);
Best Practices
Cryptographically secure random number generation is essential for any application where security matters. Both Python and Node.js provide robust, built-in modules that make it straightforward to generate secure random numbers without the need to implement complex algorithms yourself.
Here are some of the best practices to keep in mind:
- Understand Your Needs: Use standard randomness for simulations and games; use cryptographic randomness for security-related tasks.
- Do NOT Implement Your Own CSPRNG Algorithms: Creating your own cryptographic algorithms is highly discouraged unless you are a cryptography expert. Always use well-tested and proven libraries and modules instead of writing your own cryptographic functions.
- Stay Updated: Keep your programming environment and libraries up to date to benefit from security patches and improvements.
- Handle Exceptions and Errors: Always handle potential exceptions when dealing with cryptographic functions to avoid unexpected crashes or security loopholes.
- Keep Libraries Updated: Regularly update your programming environment and dependencies to benefit from security patches and improvements.
By choosing the appropriate method for generating random values, you can ensure the security and reliability of your applications.
NodeJS Error Handling Example
Here's an example of error handling in Node.js when using randomBytes():
const crypto = require("crypto");
crypto.randomBytes(16, (err, buffer) => {
if (err) {
// Handle the error securely
console.error("Error generating random bytes:", err);
return;
}
console.log("Secure Random Bytes:", buffer);
});
Final Tips for a Secure Random Password Generator
By following best practices and leveraging these tools, you can enhance the security of your applications and protect sensitive data from potential threats:
- Avoid Using Math.random(): The Math.random() function is not cryptographically secure and should not be used for security-critical applications.
- Use High-Quality Sources of Entropy: Ensure that the random number generators you use gather entropy from reliable and secure sources provided by the operating system.
Understanding the Limitations
While using built-in modules provides a high level of security, it's essential to understand their limitations:
- Performance Overhead: Cryptographically secure functions may be slower than their non-secure counterparts.
- Platform Dependency: The quality of randomness can depend on the underlying operating system.
- Random Number Range: Generating numbers within a specific range securely requires careful implementation to avoid biases.
Secure Random Crypto References
- Python Documentation
- Node.js Documentation
- Security Best Practices
- Avoid using non-secure random number generators for security-critical applications.
- Keep your systems and dependencies updated to the latest versions.
By integrating these practices into your development workflow, you ensure that your applications are both secure and reliable.
Conclusion
Randomness is a vital component in many programming tasks, but the type of randomness required varies based on the application. Standard random functions like Python's random module and JavaScript's Math.random() are suitable for non-security-critical applications where performance is essential, and predictability is not a concern.
However, in security-sensitive contexts such as generating passwords, tokens, or encryption keys, cryptographically secure randomness is indispensable. Python's secrets module and JavaScript's crypto module provide robust tools for generating secure random values.