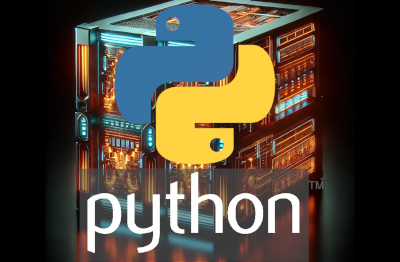
Simple HTTP Server in Python
Let's cover how to build an HTTP server in Python. We will also discuss how to Dockerize the HTTP web server using a Dockerfile based on a lightweight Python image.
DISCLAIMER: The introduction and conclusion were written by ChatGPT.
Install Python and PIP
To get started with Python, you can download the installer from the official Python website, or use a terminal to install it directly. This will allow you to set up a Python HTTP server with ease.
Install Python on Ubuntu
On Debian-based distributions like Ubuntu, installing Python is simple:
sudo apt update
sudo apt install python3
Install Python on macOS with Homebrew
Using a package manager like Homebrew simplifies the process of keeping your Python installation up to date. If you install Python from the official website, you will need to return to the site and download new versions manually whenever updates are released. However, Homebrew automates this process so that your Python environment remains current without the extra hassle.
If you're using a Mac just install the Homebrew edition of Python for macOS with the following command:
brew upgrade && brew install python@3.12
Install Setup Tools
Setuptools is essential for managing Python packages. You can upgrade it by running:
python3 -m pip install --upgrade setuptools
Install PIP
If pip isn't installed already, you can use the ensurepip flag to set it up:
Use the ensurepip flag:
python -m ensurepip --upgrade
Install pip for Windows
In a Windows command prompt, use this command to install pip:
py -m ensurepip --upgrade
Upgrade PIP
It's a good practice to keep pip up to date. Run this command to upgrade pip to the latest version:
python3 -m pip install --upgrade pip
Setup a Web Page Design
Before running the Python HTTP server, you will need a basic HTML and CSS website to serve. Below is a simple example of how to set up a basic web page that can be served using Python's HTTP service.
HTML Code for the Python HTTP Server
Here's some boilerplate, example HTML you can use to test the Python-based HTTP web server:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Test Page</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<header>
<h1>Welcome to the Test Page</h1>
</header>
<main>
<p>This is a simple HTML page to test the Python HTTP server.</p>
<p>The styles are applied from <code>style.css</code>.</p>
</main>
<footer>
<p>© 2024 Test Server</p>
</footer>
</body>
</html>
Basic CSS Template Example
We'll need some basic CSS styling for the web page as well:
body {
font-family: Arial, sans-serif;
line-height: 1.6;
margin: 0;
padding: 0;
background-color: #f4f4f4;
}
header {
background: #333;
color: #fff;
padding: 1rem;
text-align: center;
}
header h1 {
margin: 0;
}
main {
padding: 2rem;
text-align: center;
}
footer {
background: #333;
color: #fff;
padding: 1rem;
text-align: center;
position: fixed;
width: 100%;
bottom: 0;
}
Command to Start an HTTP Web Server with Python
To launch the HTTP server on port 8080 and bind it to all network interfaces (0.0.0.0), ensuring it can be accessed from any device on the network, use the following command:
python -m http.server 8080 --bind 0.0.0.0
NOTE: The default port for http.server is 8000—using the http.server 8080 syntax you can specify a custom port for Python's built-in HTTP service.
View the Python Web App
Navigate to http://localhost:8080 to view the web page.
Dockerize the Python Simple HTTP Server
If you'd like to containerize your Python HTTP server using Docker, the process is straightforward. We'll cover how to create a lightweight Docker image and how to run your HTTP server in a container.
Installing Docker
If Docker is not installed on your system, you can follow the official Docker installation documentation for the latest instructions.
Directory Structure for the Docker Project
Your Python basic HTTP server file structure should look like this:
├── Dockerfile
├── docker-compose.yml
└── website
├── index.html
└── styles.css
Docker Hub for Python
Visit the Python page on Docker Hub to view the latest Python Docker images. It's recommended to use a lightweight image such as python:slim or python:alpine for efficiency.
NOTE: It's a good idea to use a more specific image build (i.e. don't use :latest whenever possible), in order to avoid issues or bugs, in case the non-specific image does not work well with your current code or setup.
Create a Dockerfile for the Python HTTP Service
Here's an example of a Dockerfile that will run your Python HTTP service using the slim version of Python 3.12:
FROM python:3.12-slim
# Set the working directory
WORKDIR /app
# Install any necessary Python packages (e.g., if needed)
RUN pip install --no-cache-dir httpserver
# Expose the port to the world
EXPOSE 9000
# Command to run the Python HTTP server
CMD ["python3", "-m", "http.server", "9000"]
This Dockerfile defines the base image, installs necessary Python packages, and exposes port 9000 to allow access to the HTTP server. The CMD instruction specifies the command that starts the Python HTTP server when the container is launched.
Docker Run Command
After configuring the Dockerfile, you can build and run the containerized HTTP server using the following commands:
docker build -t my-python-http-server .
docker run -d -p 8080:8080 my-python-http-server
- docker build creates the Docker image with the tag my-python-http-server.
- docker run starts the container and maps port 8080 from the container to port 8080 on your host machine.
Docker Compose File
If you want to use a compose file instead, then the YAML Docker Compose file should look like this:
services:
python_web:
container_name: python_web
build: .
ports:
- "9000:9000"
volumes:
- ./website:/app
NOTE: The version line for the Docker compose file is now deprecated.
Compose Command
To build and start the Python HTTP service with Docker Compose you just run:
docker compose -f docker-compose.yml build
docker compose -f docker-compose.yml up
NOTE: If the compose file is named docker-compose.yml then you can just do docker compose build and docker compose up without having to explicitely pass the file with the -f flag.
To run the container in detached mode you can add the -d flag:
docker compose -f docker-compose.yml up -d
You should see output similar to this:
[+] Running 2/2
✔ Network python-simple-server_default Created 0.0s
✔ Container python_web Started 0.1s
Access localhost from Docker Container
Once the container is running, you can access the web server at http://localhost:9000.
Check the Docker Logs
To check the logs of your Docker Compose service, run:
docker logs python_web
To view running containers, use:
docker ps -a
It should output a table like so:
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
25c8bc238983 python-simple-server-python_web "python3 -m http.ser…" About a minute ago Up About a minute 0.0.0.0:9000->9000/tcp python_web
The -a flag will return all Docker containers—even the stopped ones:
docker stop python_web
25c8bc238983
Notice how the docker ps command still returns the container, and tells you how much time has passed since the container exited:
docker ps -a
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
25c8bc238983 python-simple-server-python_web "python3 -m http.ser…" 30 minutes ago Exited (137) 2 seconds ago python_web
The Python webserver, running inside of the Docker container, should output something like this:
192.168.65.1 - - [07/Sep/2024 08:39:28] "GET / HTTP/1.1" 200 -
192.168.65.1 - - [07/Sep/2024 08:39:28] "GET /style.css HTTP/1.1" 200 -
192.168.65.1 - - [07/Sep/2024 08:39:29] "GET / HTTP/1.1" 304 -
Conclusion
Building a simple HTTP server in Python is an excellent way to quickly serve static files like HTML, CSS, and JavaScript. Dockerizing the Python HTTP server makes it portable, easy to deploy, and simple to scale. Whether you're setting up a local development environment or preparing for production, using Python